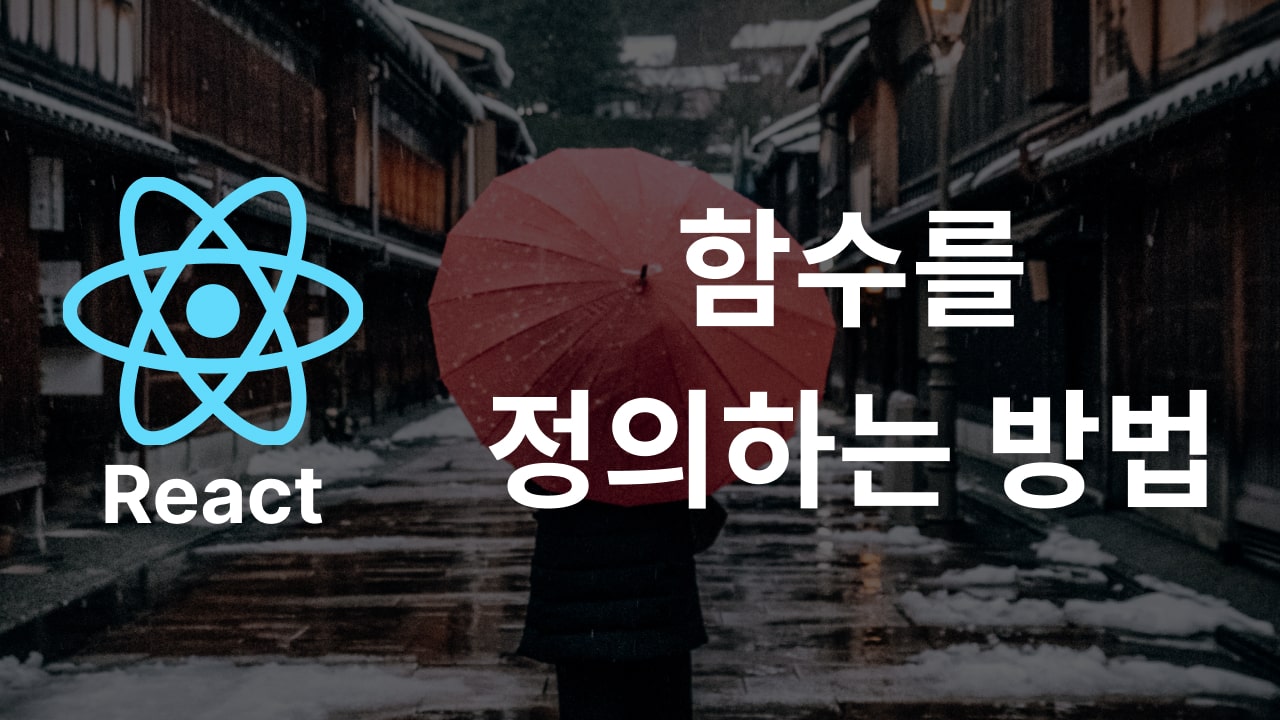
React 함수를 정의하는 방법
2023-03-23
안녕하세요.
지난 시간에는 props로 전달받은 값을 if 문으로 조건부 렌더링하는 방법에 대해서 알아보겠습니다.
이번 시간에는 함수를 정의하는 방법에 대해서 알아보겠습니다.
지난 시간에 함수를 정의할 때 아래와 같이 const를 사용해서 함수를 정의했었습니다.
<!DOCTYPE html>
<html lang="en">
<body>
<script crossorigin src="https://unpkg.com/react@18/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@18/umd/react-dom.development.js"></script>
<script crossorigin src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
<div id="root"></div>
<script type="text/babel">
const rootElement = document.getElementById("root");
const Message = ({ text }) => {
if (text.charAt(0) === text.charAt(0).toUpperCase()) {
return <h1>{text}</h1>;
} else {
return <h3>{text}</h3>;
}
};
const element = (
<>
<Message text="Chrome" />
<Message text="chrome" />
</>
);
ReactDOM.render(element, rootElement);
</script>
</body>
</html>
이번에는 함수를 정의할 때 function 키워드를 사용해서 함수를 정의해보겠습니다.
<!DOCTYPE html>
<html lang="en">
<body>
<script crossorigin src="https://unpkg.com/react@18/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@18/umd/react-dom.development.js"></script>
<script crossorigin src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
<div id="root"></div>
<script type="text/babel">
const rootElement = document.getElementById("root");
const Message = ({ text }) => {
if (text.charAt(0) === text.charAt(0).toUpperCase()) {
return <h1>{text}</h1>;
} else {
return <h3>{text}</h3>;
}
};
function Message({ text }) {
return text.charAt(0) === text.charAt(0).toUpperCase() ? <h1>{text}</h1> : <h3>{text}</h3>;
}
const element = (
<>
<Message text="Chrome" />
<Message text="chrome" />
</>
);
ReactDOM.render(element, rootElement);
</script>
</body>
</html>
위와 같이 function 키워드를 사용해서 함수를 정의할 수 있습니다.
function 키워드를 사용해서 함수를 정의할 때는 function 키워드 뒤에 함수의 이름을 적어주고, 함수의 매개변수를 정의해주고, 함수의 내용을 정의해주면 됩니다.
이렇게 const 키워드를 사용해서 함수를 정의할 수도 있고, function 키워드를 사용해서 함수를 정의할 수도 있습니다.
감사합니다.
< 목록으로 돌아가기